C# WinForms 应用中使用嵌入资源(Embedded Resources)
|
admin
2024年10月8日 9:9
本文热度 215
|
在 C# Windows Forms (WinForms) 应用开发中,经常会遇到需要将文件嵌入到程序集中的情况。这些文件可以是图像、文档、音频、视频或任何其他类型的文件。通过将这些文件作为嵌入资源(Embedded Resources)包含在程序集中,可以简化应用的部署和分发,确保这些资源始终可用,且不易丢失或被篡改。
应用场景
图像和图标:将应用程序使用的图标、按钮图像、背景图像等嵌入到程序集中。
配置文件:将配置文件嵌入程序集,避免外部配置文件丢失或被错误修改。
本地化资源:存储多语言文本、图像等资源,支持应用的国际化。
帮助文件和文档:将用户手册、帮助文档等嵌入程序集,方便用户访问。
音频和视频文件:嵌入音频和视频文件,用于应用中的多媒体播放。
如何嵌入文件
在 Visual Studio 的解决方案资源管理器中,将文件添加到 WinForms 项目中。
选中文件,在“属性”窗口中将“生成操作”设置为“嵌入的资源”。
示例
示例 1:显示嵌入的图像
假设我们将一个名为 logo.png
的图像文件嵌入到 WinForms 应用程序中。
using System;
using System.Drawing;
using System.Reflection;
using System.Windows.Forms;
public class MainForm : Form
{
private PictureBox pictureBox;
public MainForm()
{
pictureBox = new PictureBox
{
Size = new Size(200, 200),
Location = new Point(10, 10)
};
this.Controls.Add(pictureBox);
LoadEmbeddedImage();
}
private void LoadEmbeddedImage()
{
var assembly = Assembly.GetExecutingAssembly();
var resourceName = "YourNamespace.logo.png";
using (var stream = assembly.GetManifestResourceStream(resourceName))
{
pictureBox.Image = Image.FromStream(stream);
}
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
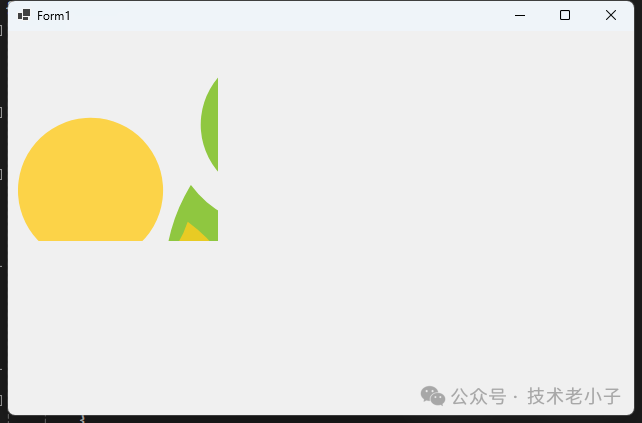
示例 2:读取嵌入的文本配置文件
假设我们有一个名为 config.txt
的文本配置文件,我们将其嵌入到 WinForms 应用程序中。
using System;
using System.IO;
using System.Reflection;
using System.Windows.Forms;
public class MainForm : Form
{
public MainForm()
{
string configData = LoadEmbeddedTextFile("YourNamespace.config.txt");
MessageBox.Show(configData, "Config Data");
}
private string LoadEmbeddedTextFile(string resourceName)
{
var assembly = Assembly.GetExecutingAssembly();
using (var stream = assembly.GetManifestResourceStream(resourceName))
using (var reader = new StreamReader(stream))
{
return reader.ReadToEnd();
}
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
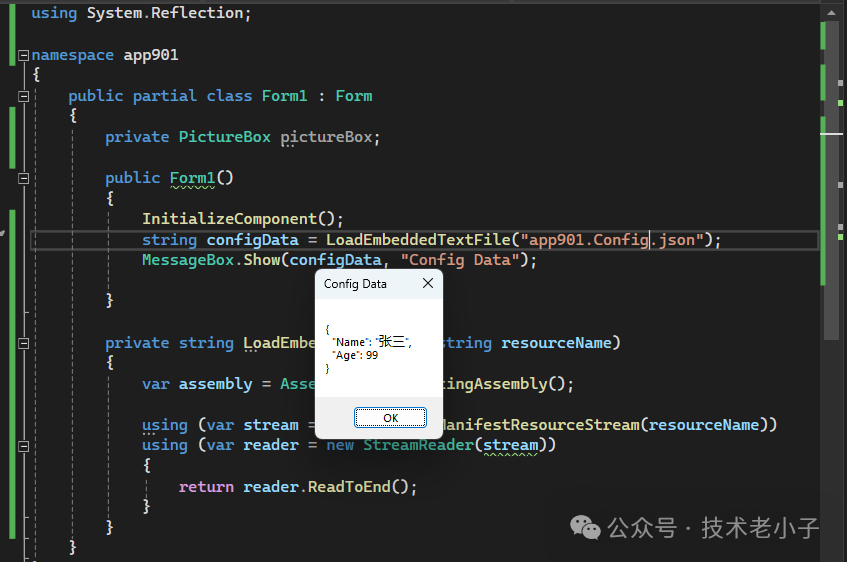
注意:YourNamespace.config.txt 区分大小写
示例 3:播放嵌入的音频文件
假设我们将一个名为 sound.mp3
的音频文件嵌入到 WinForms 应用程序中,并使用 System.Media.SoundPlayer
或第三方库来播放它。
using System;
using System.IO;
using System.Media;
using System.Reflection;
using System.Windows.Forms;
public class MainForm : Form
{
public MainForm()
{
Button playButton = new Button
{
Text = "Play Sound",
AutoSize = true,
Location = new Point(10, 10)
};
playButton.Click += (sender, e) => PlayEmbeddedSound("YourNamespace.sound.mp3");
this.Controls.Add(playButton);
}
private void PlayEmbeddedSound(string resourceName)
{
var assembly = Assembly.GetExecutingAssembly();
using (var stream = assembly.GetManifestResourceStream(resourceName))
{
var soundPlayer = new SoundPlayer(stream);
soundPlayer.Play();
}
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
结论
将文件作为嵌入资源包含在 C# WinForms 应用程序的程序集中,提供了一种有效的方法来管理和访问这些资源。这种方法简化了应用程序的部署和分发过程,确保资源始终可用,并减少了文件丢失或被篡改的风险。上述示例展示了如何在 WinForms 应用中读取和使用不同类型的嵌入资源,从而为开发者提供了实用的参考。
该文章在 2024/10/8 20:37:38 编辑过